Creating your first Tarantool Cartridge application
Here we’ll walk you through developing a simple cluster application.
First, set up the development environment.
Next, create an application named myapp
. Run:
$ cartridge create --name myapp
This will create a Tarantool Cartridge application in the ./myapp
directory,
with a handful of
template files and directories
inside.
Go inside and make a dry run:
$ cd ./myapp
$ cartridge build
$ cartridge start
This will build the application locally, start 5 instances of Tarantool and a stateboard (state provider), and run the application as it is, with no business logic yet.
Why 5 instances and a stateboard? See the instances.yml
file in your application directory.
It contains the configuration of all instances
that you can use in the cluster. By default, it defines configuration for 5
Tarantool instances and a stateboard.
---
myapp.router:
advertise_uri: localhost:3301
http_port: 8081
myapp.s1-master:
advertise_uri: localhost:3302
http_port: 8082
myapp.s1-replica:
advertise_uri: localhost:3303
http_port: 8083
myapp.s2-master:
advertise_uri: localhost:3304
http_port: 8084
myapp.s2-replica:
advertise_uri: localhost:3305
http_port: 8085
myapp-stateboard:
listen: localhost:4401
password: passwd
You can already see these instances in the cluster management web interface at
http://localhost:8081 (here 8081 is the HTTP port of the first instance
specified in instances.yml
).

Okay, press Ctrl + C
to stop the cluster for a while.
Now it’s time to add some business logic to your application. This will be an evergreen “Hello world!”” – just to keep things simple.
Rename the template file app/roles/custom.lua
to hello-world.lua
.
$ mv app/roles/custom.lua app/roles/hello-world.lua
This will be your role. In Tarantool Cartridge, a role is a Lua module that implements some instance-specific functions and/or logic. Further on we’ll show how to add code to a role, build it, enable and test.
There is already some code in the role’s init()
function.
local function init(opts) -- luacheck: no unused args
-- if opts.is_master then
-- end
local httpd = assert(cartridge.service_get('httpd'), "Failed to get httpd service")
httpd:route({method = 'GET', path = '/hello'}, function()
return {body = 'Hello world!'}
end)
return true
end
This exports an HTTP endpoint /hello
. For example, http://localhost:8081/hello
if you address the first instance from the instances.yml
file.
If you open it in a browser after enabling the role (we’ll do it here a bit later),
you’ll see “Hello world!” on the page.
Let’s add some more code there.
local function init(opts) -- luacheck: no unused args
-- if opts.is_master then
-- end
local httpd = cartridge.service_get('httpd')
httpd:route({method = 'GET', path = '/hello'}, function()
return {body = 'Hello world!'}
end)
local log = require('log')
log.info('Hello world!')
return true
end
This writes “Hello, world!” to the console when the role gets enabled, so you’ll have a chance to spot this. No rocket science.
Next, amend role_name
in the “return” section of the hello-world.lua
file.
You’ll see this section at the bottom of the file.
This text will be displayed as a label for your role in the cluster management
web interface.
return {
role_name = 'Hello world!',
init = init,
stop = stop,
validate_config = validate_config,
apply_config = apply_config,
-- dependencies = {'cartridge.roles.vshard-router'},
}
The final thing to do before you can run the application is to add your role to
the list of available cluster roles in the init.lua
file in the project root directory.
local cartridge = require('cartridge')
local ok, err = cartridge.cfg({
roles = {
'cartridge.roles.vshard-storage',
'cartridge.roles.vshard-router',
'cartridge.roles.metrics',
'app.roles.hello-world',
},
})
Now the cluster will be aware of your role.
Why app.roles.hello-world
? By default, the role name here should match the
path from the application root (./myapp
) to the role file
(app/roles/hello-world.lua
).
Great! Your role is ready. Re-build the application and re-start the cluster now:
$ cartridge build
$ cartridge start
Now all instances are up, but idle, waiting for you to enable roles for them.
Instances (replicas) in a Tarantool Cartridge cluster are organized into replica sets. Roles are enabled per replica set, so all instances in a replica set have the same roles enabled.
Let’s create a replica set containing just one instance and enable your role:
Open the cluster management web interface at http://localhost:8081.
Next to the router instance, click Configure.
Check the role
Hello world!
to enable it. Notice that the role name here matches the label text that you specified in therole_name
parameter in thehello-world.lua
file.(Optionally) Specify the replica set name, for example “hello-world-replica-set”.
Click Create replica set and see the newly-created replica set in the web interface.
Your custom role got enabled. Find the “Hello world!” message in console, like this:

Finally, open the HTTP endpoint of this instance at http://localhost:8081/hello and see the reply to your GET request.
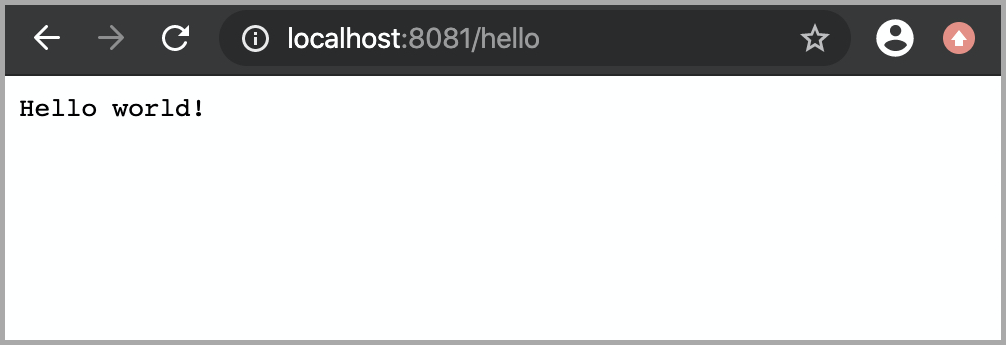
Everything is up and running! What’s next?
- Follow the administrator’s guide to set up the rest of the cluster and try some cool cluster management features – for example, enable failover.
- Check out the Cartridge developer’s guide and implement more sophisticated business logic for your role.
- Pack your application for easy distribution. Choose what you like: a DEB or RPM package, a TGZ archive, or a Docker image. Archives and packages can be deployed with ansible-cartridge.
- Read the Cartridge documentation.